destroy and mount datatable component
destroy and mount datatable component

I have this standard component
<script setup lang="ts">
import DataTable from 'datatables.net-vue3'
import DataTablesCore from 'datatables.net'
import 'datatables.net-select'
import 'datatables.net-responsive'
DataTable.use(DataTablesCore)
const emit = defineEmits(['editAction', 'deleteAction'])
const props = defineProps({
data: { type: Array as () => any[], required: true },
columns: { type: Array as () => any[], required: true },
tableId: { String, required: true },
editAction: { type: Function, required: true },
deleteAction: { type: Function, required: true }
})
const columns = [
...props.columns,
{
data: null,
render: '#edit',
title: 'Editar',
width: '2%',
orderable: false,
searchable: false
},
{
data: null,
render: '#delete',
title: 'Deletar',
width: '2%',
orderable: false,
searchable: false
}
]
const tableOptions = {
options: {
buttons: ['copy'],
destroy: true,
// select: true,
processing: false,
responsive: {
reponsive: true,
breakpoints: [
{ name: 'bigdesktop', width: Infinity },
{ name: 'meddesktop', width: 1480 },
{ name: 'smalldesktop', width: 1280 },
{ name: 'medium', width: 1188 },
{ name: 'tabletl', width: 1024 },
{ name: 'btwtabllandp', width: 848 },
{ name: 'tabletp', width: 768 },
{ name: 'mobilel', width: 480 },
{ name: 'mobilep', width: 320 }
]
},
language: {
search: 'Pesquisar:',
lengthMenu: 'Mostrar _MENU_ registros por página',
info: 'Mostrando _START_ até _END_ de _TOTAL_ registros',
infoEmpty: 'Nenhum registro encontrado',
emptyTable: 'Nenhum registro encontrado',
infoFiltered: '(filtrado de _MAX_ registros no total)',
zeroRecords: 'Nenhum registro corresponde aos critérios de pesquisa',
select: {
rows: {
_: ' %d linhas selecionadas',
0: '',
1: ''
}
},
paginate: {
first: 'Primeiro',
previous: 'Anterior',
next: 'Próximo',
last: 'Último'
}
}
}
}
function handleEdit(rowData: any) {
emit('editAction', rowData)
}
function handleDelete(rowData: any) {
emit('deleteAction', rowData)
}
</script>
<template>
<DataTable
ref="dataTable"
:tableId="props.tableId"
:data="props.data"
:columns="columns"
:options="tableOptions.options"
class="display w-full table-auto"
width="100%"
>
<template #edit="props">
<div class="flex items-center justify-center">
<button @click="handleEdit(props.rowData)">
<v-icon name="fa-edit" scale="1" title="Editar" />
</button>
</div>
</template>
<template #delete="props">
<div class="flex items-center justify-center">
<button @click="handleDelete(props.rowData)">
<v-icon name="fa-trash" scale="1" title="Deletar" />
</button>
</div>
</template>
</DataTable>
</template>
<style>
@import 'datatables.net-dt';
@import 'datatables.net-responsive-dt';
#DataTables_Table_0_wrapper {
background-color: white;
border-radius: 4px;
margin-top: 40px;
}
#DataTables_Table_0_wrapper .dt-search,
#DataTables_Table_0_wrapper .dt-length,
#DataTables_Table_0_wrapper .dt-paging,
#DataTables_Table_0_wrapper .dt-info {
padding: 20px 20px;
}
#DataTables_Table_0_wrapper .dt-paging .dt-paging-button.current {
}
#DataTables_Table_0 .dt-empty {
text-align: center;
}
#DataTables_Table_0 thead tr th {
text-align: left;
}
#DataTables_Table_0 tbody tr td {
text-align: left;
}
</style>
and when leaving the view, I need to disassemble and when returning, mount the component
and I call it ana view that I need, passing the data
<DataTable
:data="tableData"
:columns="tableColumns"
:table-id="empregadoresTable"
@editAction="editEmpregador"
:editAction="editEmpregador"
@deleteAction="openModalDelete"
:deleteAction="openModalDelete"
/>
Edited by Colin - Syntax highlighting. Details on how to highlight code using markdown can be found in this guide.
Replies
Sorry, I'm not clear what the issue is - are you see errors or problems?
Colin
When I change views, the CSS is broken and it only works again when the page is reloaded.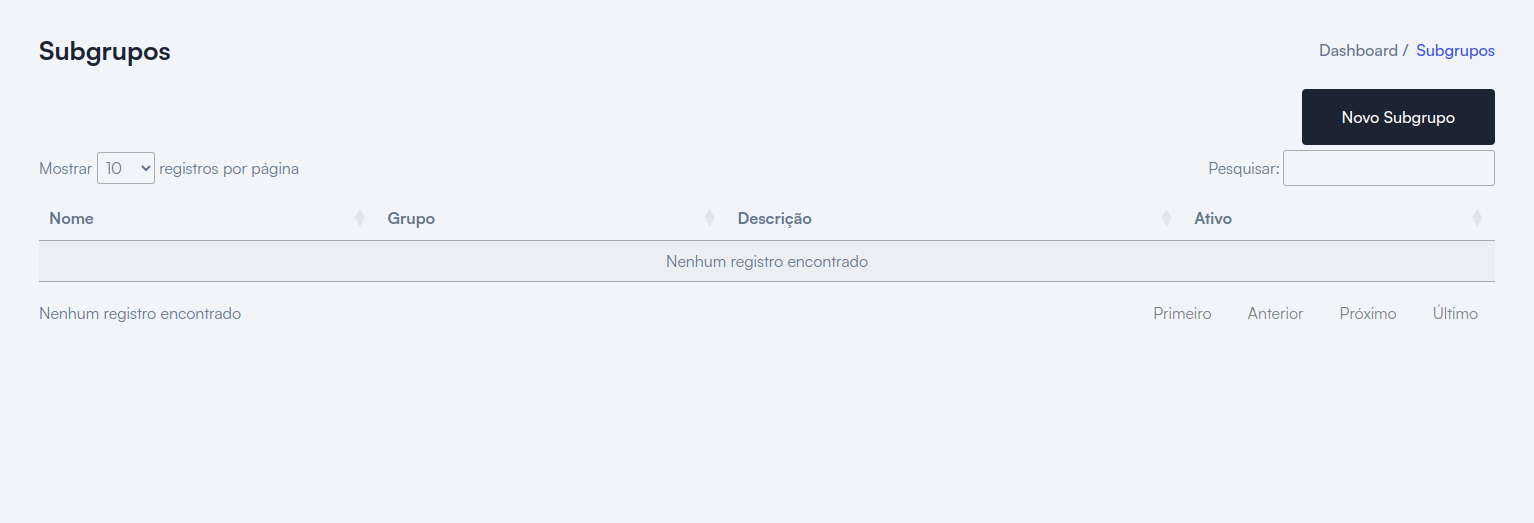
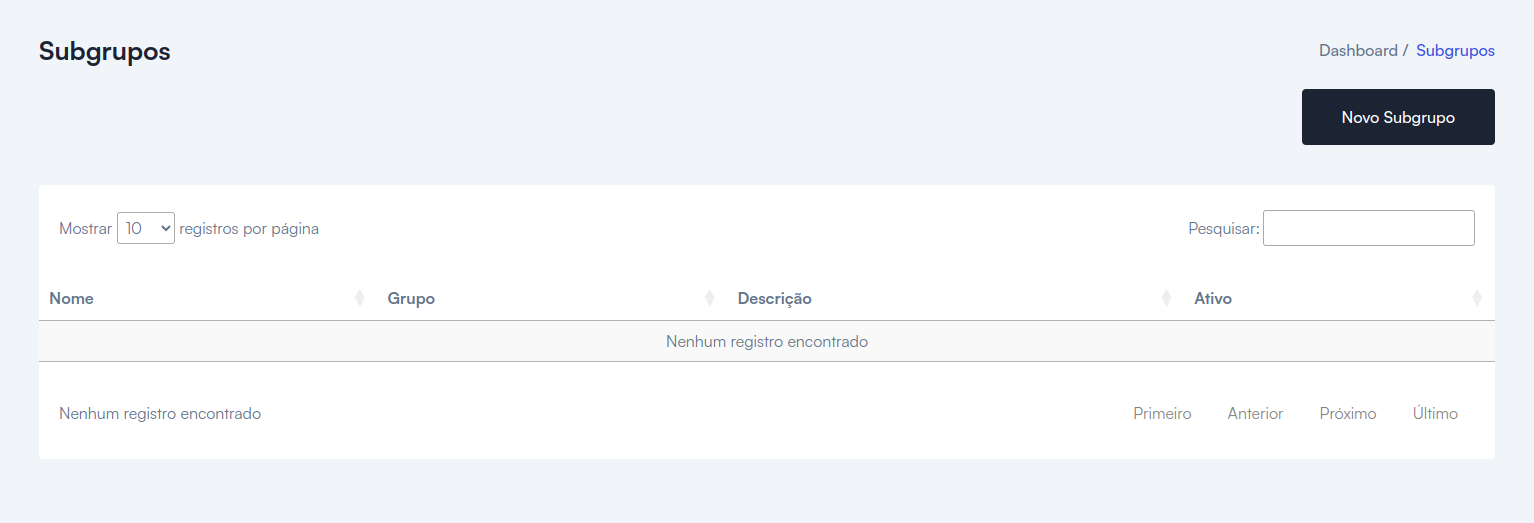
We're happy to take a look, but as per the forum rules, please link to a test case - a test case that replicates the issue will ensure you'll get a quick and accurate response. Information on how to create a test case (if you aren't able to link to the page you are working on) is available here.
Colin
StackBltiz is great for test cases of this sort of nature. You could perhaps use this example as the basis for building up an example showing the issue.
I wonder if the CSS is being loaded multiple times, but that would see an odd thing to happen - I wouldn't expect that in Vue.
Allan